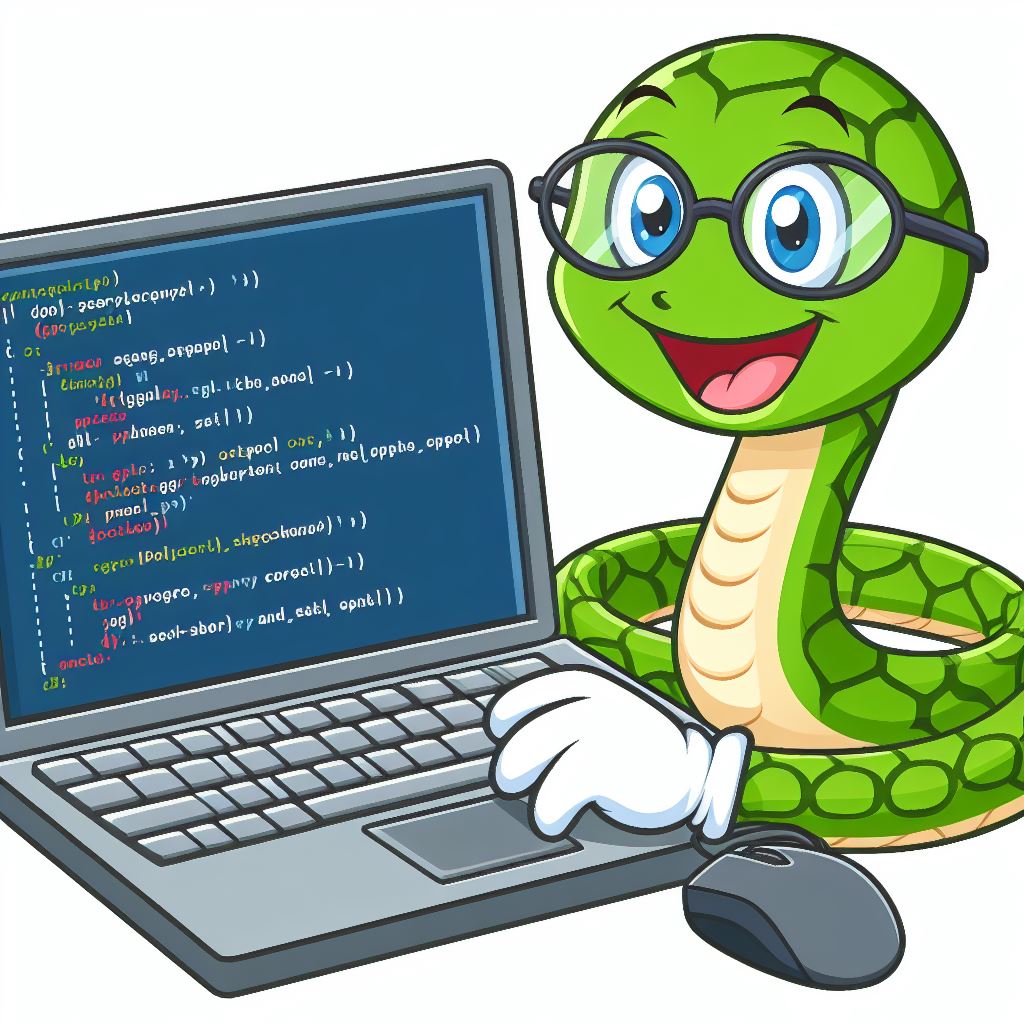
Pseudo Code
The purpose of pseudo code is to facilitate the communication of ideas and explanations in documentation and discussions. It is a fictious programming language and no real working software is produced in it. The concepts expressed in pseudo code can however be converted into real functioning code by a programmer.
There are a large number of programming languages and programmers often only know one or a few of them to a high standard. Someone wanting to write down how an algorithm works in a general sense may have to pick a language in which to write the description. The choice of language may not suit everybody and only be comprehensible to a small audience. A technical author may instead choose pseudo code as an alternative, which is often written at a high level with common concepts familiar to most programmers.
Not all members of a software team may understand how to write code and neither may the ultimate customer of the software. Pseudo code can be clearer for non-technical team members to follow than actual real working code as it eliminates anything not essential to the concept being explained.
Pseudo code may look something like this, which describes a function to calculate the average of two numbers. This style of pseudo code is somewhat reminiscent of, although not identical to, the BASIC programming language.
FUNCTION average(a: NUMBER, b: NUMBER) RETURNS NUMBER
SET sum = a + b
SET average = sum / 2
RETURN average
END FUNCTION
- On the first line we start by defining a function called “average” that accepts two numbers, a and b, and returns a number as the result.
- Next we add a and b and put the result into the variable sum
- Then we divide the sum by 2 and put the result into average.
- We return average as the final result of the function
As it is not a real language, there is no formal definition of pseudo code and many variations in the notation are seen as the author prefers. For example, you might see the function written like this instead:
FUNCTION average(a: NUMBER, b: NUMBER) : NUMBER
DECLARE sum : NUMBER
DECLARE average : NUMBER
sum ← a + b
average ← sum / 2
RETURN average
END FUNCTION
The style can vary with the educational background of the author. For example a mathematician may lean towards different notation to a computer scientist that is more familiar is mathematical circles.
If you are asked to answer exam questions in pseudo code, it is likely you will be required to adhere to a specific a style. You should be provided with an explanation of the conventions your examiner prefers to see in advance.
Some sections of a program may be quite verbose to document and irrelevant to what is being explained. Sometimes part of a program may be quite machine or operating system specific but the programmer wants to express an idea in a generic way that every reader can understand. In which case an author might mix in English words with programming-like statements or they may refer to functions with descriptive English names but provide no explanation as to how those functions work if it is not important.
For example in this code, the author may be trying to explain that a function displays a user selectable number of book covers in sequence on the screen. Exactly how the book cover photo is acquired and displayed may be complex and is not relevant to what the author is communicating and so this complexity is hidden with a procedure called “displayImage”. The exact mechanics of how this procedure works are never explained but the name implies what the procedure must do.
FUNCTION displayBookCovers(bookIDStart: NUMBER, numberOfBooksToDisplay: NUMBER)
FOR i = 0 TO numberOfBooksToDisplay
CALL displayImage( bookIDStart + i )
NEXT i
END FUNCTION