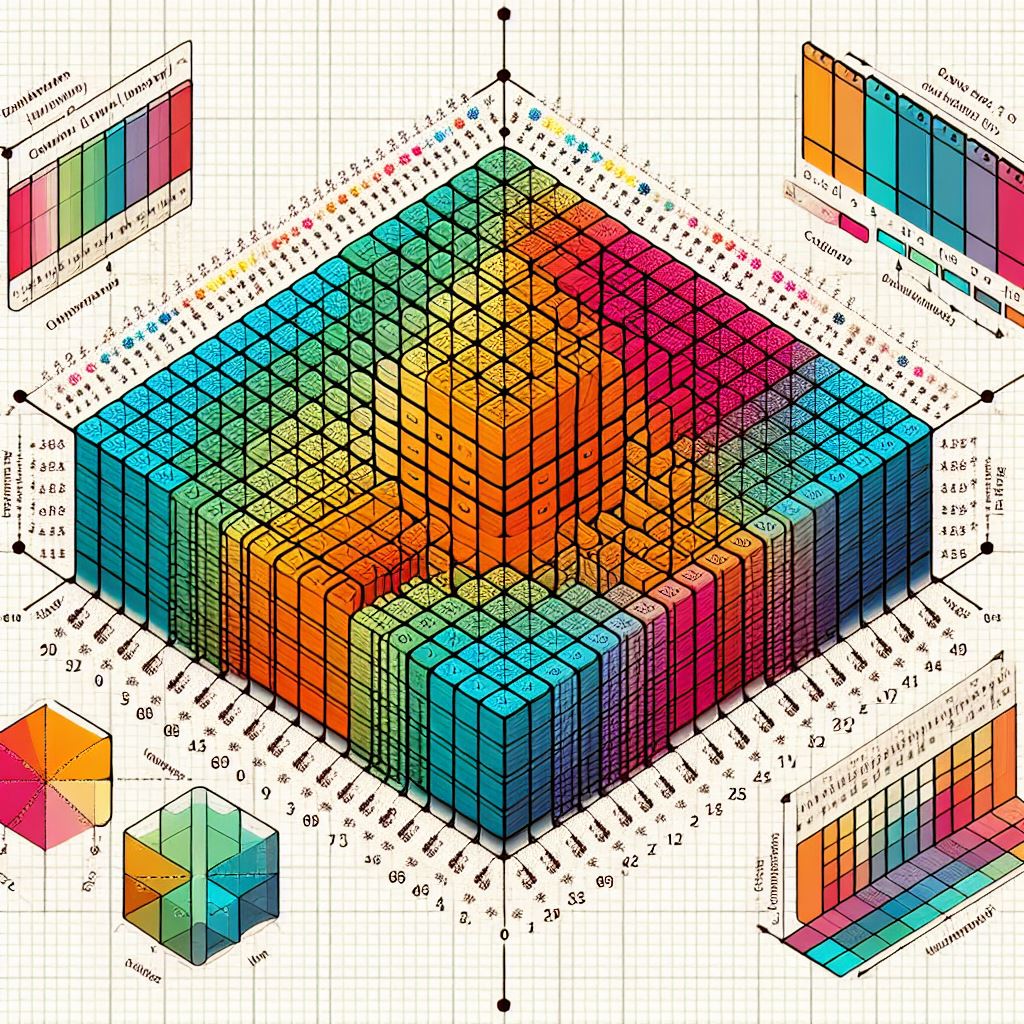
Working with Numpy Arrays in Python
Numpy arrays are commonly encountered in Python, particularly in AI and other scientific work. This guide shows a number of examples of how numpy arrays can be manipulated.
Importing Numpy
import numpy as np
Convert a Regular Python List to a Numpy Array
>>> x = (0,1,2,3,4,5,6,7,8,9,10)
>>> a_numpy_array = np.array(x)
And convert it back again to a regular Python list:
regular_list = a_numpy_array.tolist()
Fill a Numpy Array with Sequential Digits
np.arange(12)
Result:
array([ 0, 1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11])
Now create an array between 100 and 120, counting in steps of 2:
>>> np.arange(100,120,2)
array([100, 102, 104, 106, 108, 110, 112, 114, 116, 118])
Rearrange a Numpy Array from 1D to 2D and Back Again
Generate a 1D array of 12 integers and then re-arrange the 1D array into a 2D array with 4 rows and 3 columns
>>> a = np.arange(12)
>>> b = a.reshape(4,3)
>>> b
array([[ 0, 1, 2],
[ 3, 4, 5],
[ 6, 7, 8],
[ 9, 10, 11]])
Now re-arrange the 4x3 2D array back into the original 1D array of 12 integers:
>>> c = b.reshape(1,12)
>>> c
array([[ 0, 1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11]])
The 4 rows x 3 columns 2D array can be re-arranged into 3 rows x 4 columns:
>> d = b.reshape(3,4)
>>> d
array([[ 0, 1, 2, 3],
[ 4, 5, 6, 7],
[ 8, 9, 10, 11]])
Add an Extra Column to a 2D Numpy Array
>>> my_numpy_array
array([[ 0, 1, 2, 3],
[ 4, 5, 6, 7],
[ 8, 9, 10, 11]])
>>> extra_column = np.array( [100,200,300] ).reshape(3,1)
>>> extra_column
array([[100],
[200],
[300]])
>>> np.column_stack( ( my_numpy_array, extra_column ) )
array([[ 0, 1, 2, 3, 100],
[ 4, 5, 6, 7, 200],
[ 8, 9, 10, 11, 300]])
Extract a Column from a 2D Numpy Array
Extract the 2nd column from the following two dimensional numpy array:
>> my_numpy_array,
(array([[ 0, 1, 2, 3],
[ 4, 5, 6, 7],
[ 8, 9, 10, 11]]),)
>>> my_numpy_array[:,1]
array([1, 5, 9])