The Difference Between Imperative and Declarative Programming
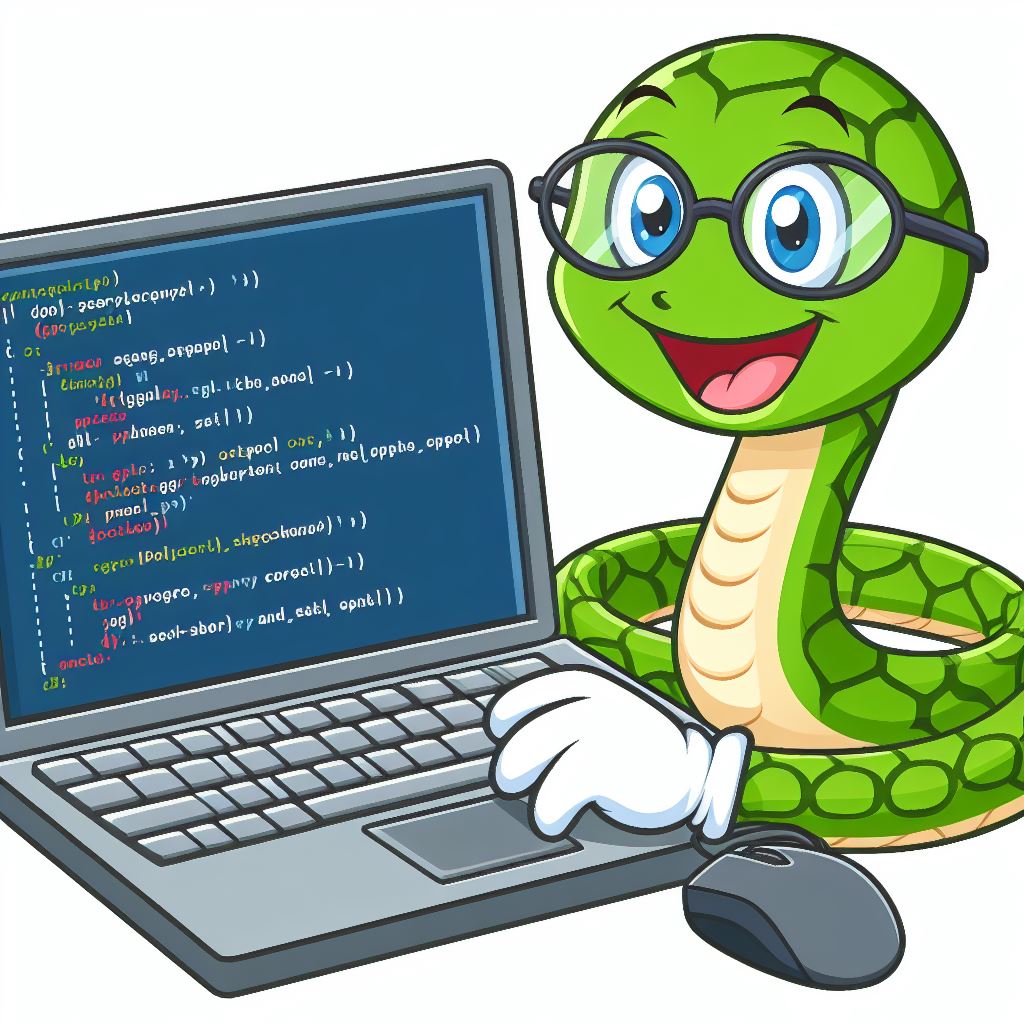
Imperative programming is the style of language (or paradigm) most commonly encountered. The programmer writes a list of instructions and the computer executes the instructions in sequence. The programmer needs to break down the user’s requirement into a series of minute individul steps that describe in detail every stage of what needs to be done.
Imperative programming languages include Java, Python and C.
In Declarative programming the programmer doesn’t state the exact sequence of steps. The programmer instead describes the desired outcome and the programming language determines the sequence of steps that needs to be executed to achieve it. Declarative programming languages include Prolog and SQL.
In SQL the programmer might write a statement like this to instruct a database:
SELECT SUM(sale_value) FROM sales WHERE region = "France";
This statement says the programmer wants to know the sum total of all sales in France. It does not state how the database will achieve this. All it states is the outcome the programmer requires. It is up to the SQL interpreter to work out what needs to be done.
In an imperative language the programmer may need to have told the database:
- Open a file of sales transactions
- Loop through them all
- Check if each transaction is from France
- If so accumulate the sale value into a variable
- Print the variable where the sales have been totalled
In declarative programming the language itself determines that sequence of steps.
Declarative programming languages can be useful in some situations. In the above example the SQL statement is more succinct than the imperative version and hides the technical details of the database. The database may have an index for example, but the SQL statement does not require the programmer to understand that the index is available, that it may be applicable to the requirement or how it should be used. SQL will use the index of its own accord if it thinks it is appropriate to do so.
Sometimes the sequence of steps is difficult to determine and this is where declarative languages can come into their own. Prolog is a language that is good at solving logic problems. You tell it everything that is known about a problem, give it a question, and then it attempts to determine if it is possible to give an answer based on the known facts.
For example in one classic logic problem, you are on an island where everyone is a knight or a knave. Knights always tell the truth and knaves always lie. You want to sort out who is who. You meet two inhabitants of the island and one of them unhelpfully reveals: “either I am a knave or the other person is a knight.” Someone experienced in logic problems can deduce that both of the people are knights. The sequence of steps needed to do this though is perhaps not entirely obvious to the rest of us and programming this in an imperative language could be tricky. Prolog can automatically reason this kind of puzzle and work out the answer. The programmer needs only to state what is known about the problem and the language is responsible for considering how to solve it.
Some business problems can be reduced to logic puzzles. For example room scheduling problems come with a number of constraints that must be resolved. Room 1 seats 8 but is only available on Tuesdays. Room 2 seats 4 and has a large screen display. Room 3 seats 10 but does not have wi-fi. Room 4 seats 6 and is a long way away. Employee A wants a room on a Wednesday for 6 people that has wi-fi. Employee B wants a room on Tuesday for two people with a large screen display. Employees prefer rooms that are closer and it is wise to keep rooms with special resources free where possible in case someone needs them later. When it gets up to hundreds of rooms and thousands of employees, it is a very complex problem to resolve the constraints. In a language like Prolog the facts about the rooms can be filled in and then the customer requirements presented. The language can determine which room, if any, best meets the requirements.
Declarative languages normally fill specific niches where determining the exact sequence of steps necessary to solve a problem is complex. These languages have a relationship to AI which performs a similar task and are sometimes known as symbolic AI.
This kind of language can be be much more difficult to debug than imperative code as it’s not always clear what the language is doing. Due to the very nature of the language there is little control over how it will solve the problem. It may for example use excessive memory in producing a solution and there is little the programmer can do about it. Where the necessary sequence of steps is simpler to reason, an imperative language will often be easier. Declarative languages are often used in tandem with imperative code to solve part of the problem but more rarely are entire systems built in them.