Controlling LIFX Smart Light Bulbs using Python
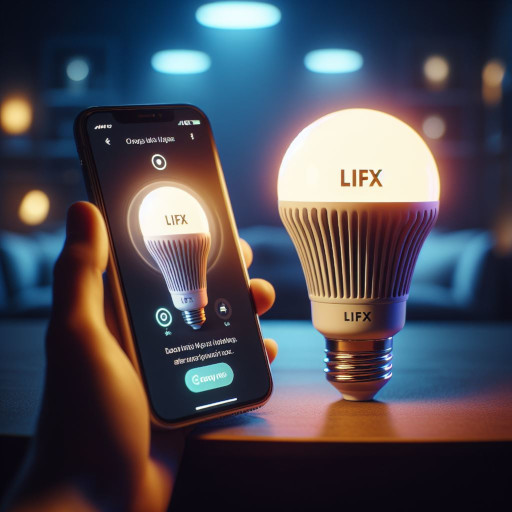
LIFX smart light bulbs can be controlled entirely on the local wi-fi network without requiring use of any cloud services.
The following Python example uses the LifxLAN module to perform the following actions:
- Scans the local network and lists the names of all of the LIFX bulbs found
- Turns the bulb called “Bedroom” on
- Changes the colour of the bulb to soft white
- Turns the bulb off
from lifxlan import LifxLAN
import time
if __name__ == "__main__":
#Enable us to refer to light objects by name
light_list = dict()
lan = LifxLAN()
#Scan the local network and enumerate the list of available LifX bulbs
for light in lan.get_lights():
if light.is_light():
light_name = str(light.get_label())
light_list[ light_name ] = light
print(f'Found light {light_name}')
#Turn the bedroom light on
light_list["Bedroom"].set_power(1);
time.sleep(3)
#Set the bedroom light to a warm white colour at 50% brightness
#Parameters: hue (range: 0-65535), saturation (range: 0-65535), brightness (range: 0-65535), Kelvin (range: 2500-9000)
light_list["Bedroom"].set_color( (0, 0, 32768, 3200) )
time.sleep(3)
#Turn the bedroom light off
light_list["Bedroom"].set_power(0);
Errors
Note, that at the time of the writing the version of the LifxLAN module in PyPi (1.2.7) seems to have a bug in it. This appears to be due to a change in the module “bitstring” which introduced breaking changes in version 4.
This results in the following exception:
bitstring.exceptions.CreationError: Token with length 64 packed with value of length 0.
A solution is to rollback to a version of bitstring that is less than version 4.
pip install bitstring==3.1.9
pip install LifxLAN