Negative Numbers in Binary: Two's Complement
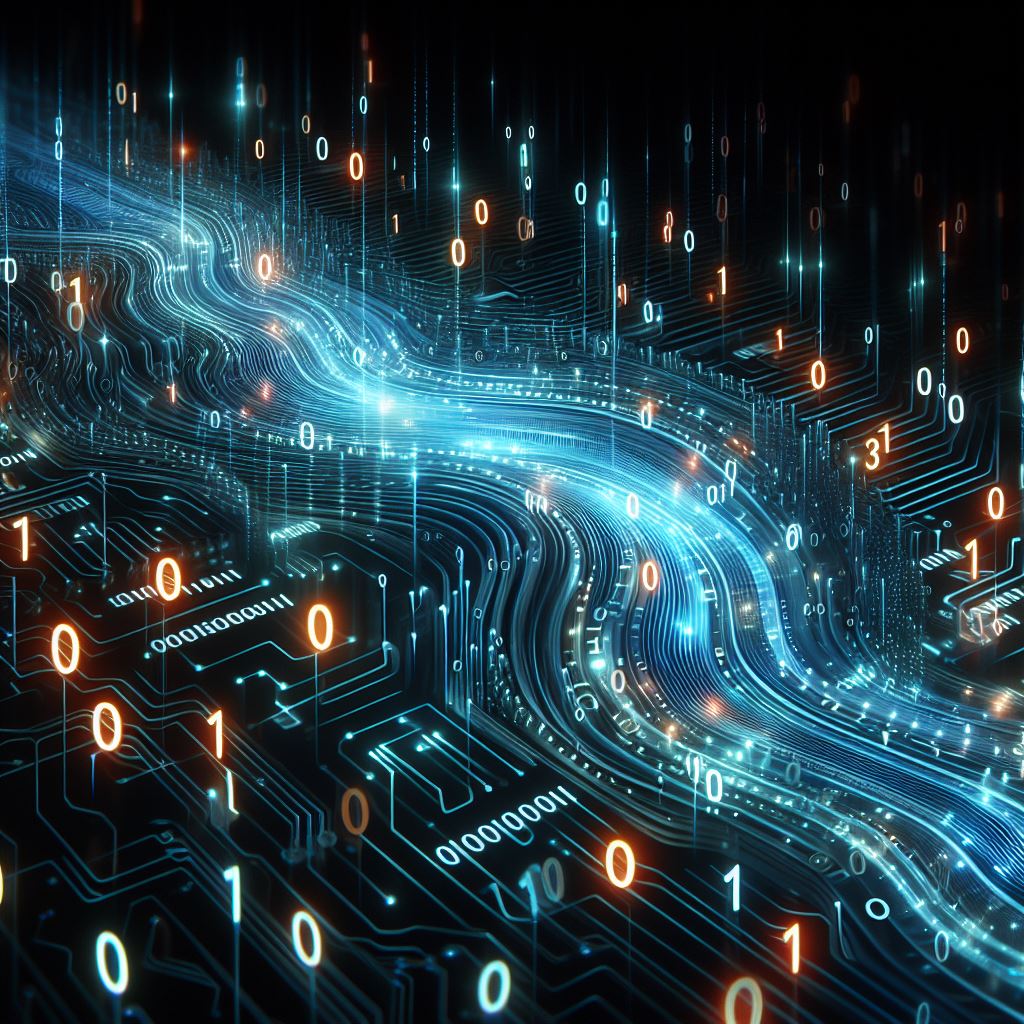
In an 8-bit byte, there are 256 possible combinations of ones and zeros. When dealing with positive integers (whole numbers) we normally use the 256 possible combinations to represent the numbers zero through 255 (one bit pattern for each number).
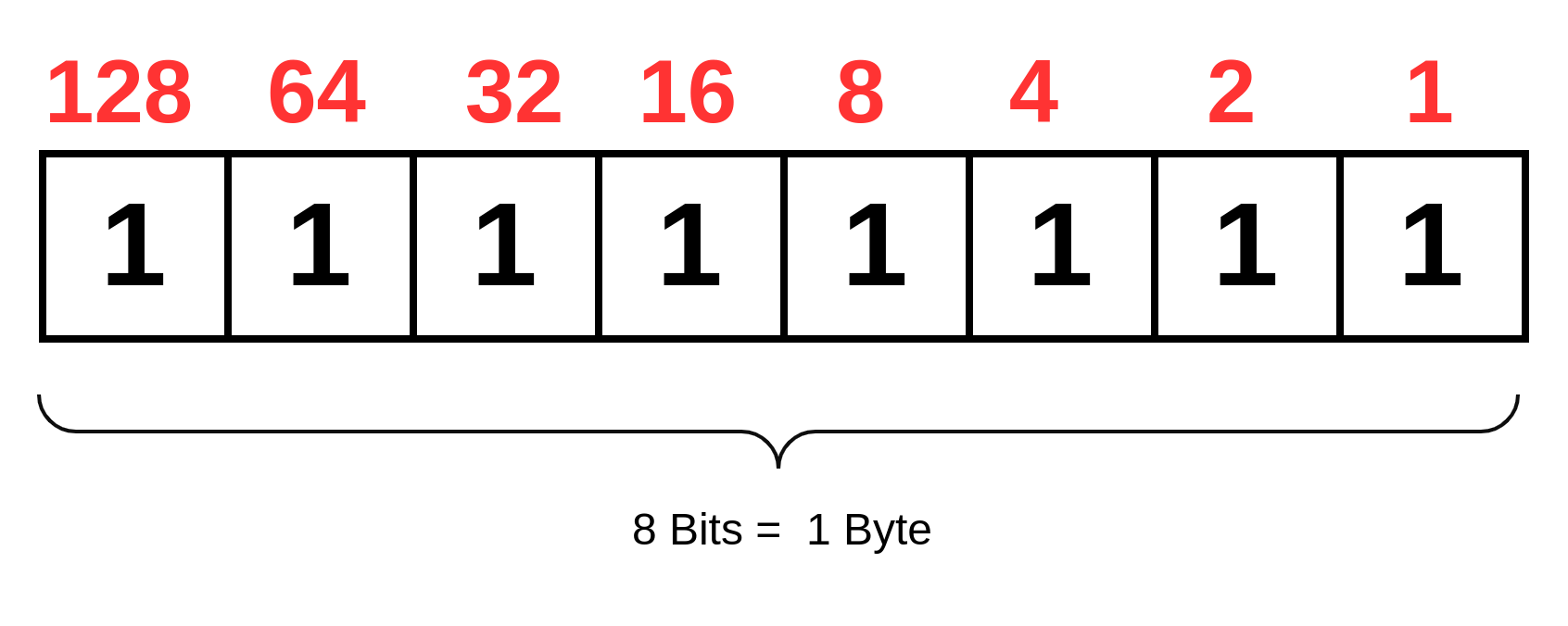
What happens though if we want to represent negative numbers? Well, we don’t have to use all of the 256 possible combinations of bits to represent positive numbers, we could use some of those combinations to represent negative numbers as well and this is exactly what is done to solve the problem. The trade-off is the more of the limited available combinations we use to represent negative numbers then the fewer combinations are available to represent positive numbers.
A popular method for representing negative numbers in binary is called “two’s complement” and this technique is used in almost all computers. Two’s complement assigns 128 of the available bit patterns to negative numbers, 127 of the bit patterns to positive numbers, and then we have one left over for zero. That is 128 negative combinations + 127 positive combinations + one pattern for zero = all of the 256 possible combinations of bits. Hence in two’s complement, using only 8-bits, we can represent numbers from -128 through +127. If we want to represent a wider range of numbers then we need more bits.
Quite often in programming, we only want to represent positive numbers and don’t care about negatives. However, when using two’s complement we lose half of the range of positives. For this reason, many programming languages offer a choice as to whether you want to allow use of negatives. The language used to describe this choice is whether you would like “signed” or “unsigned” variables.
Positive numbers in two’s complement are represented in the usual way. To convert a positive number into a negative number, we flip (invert) all the bits and then add one. For example, say we start with +17 and we want to convert it to -17, we do this:
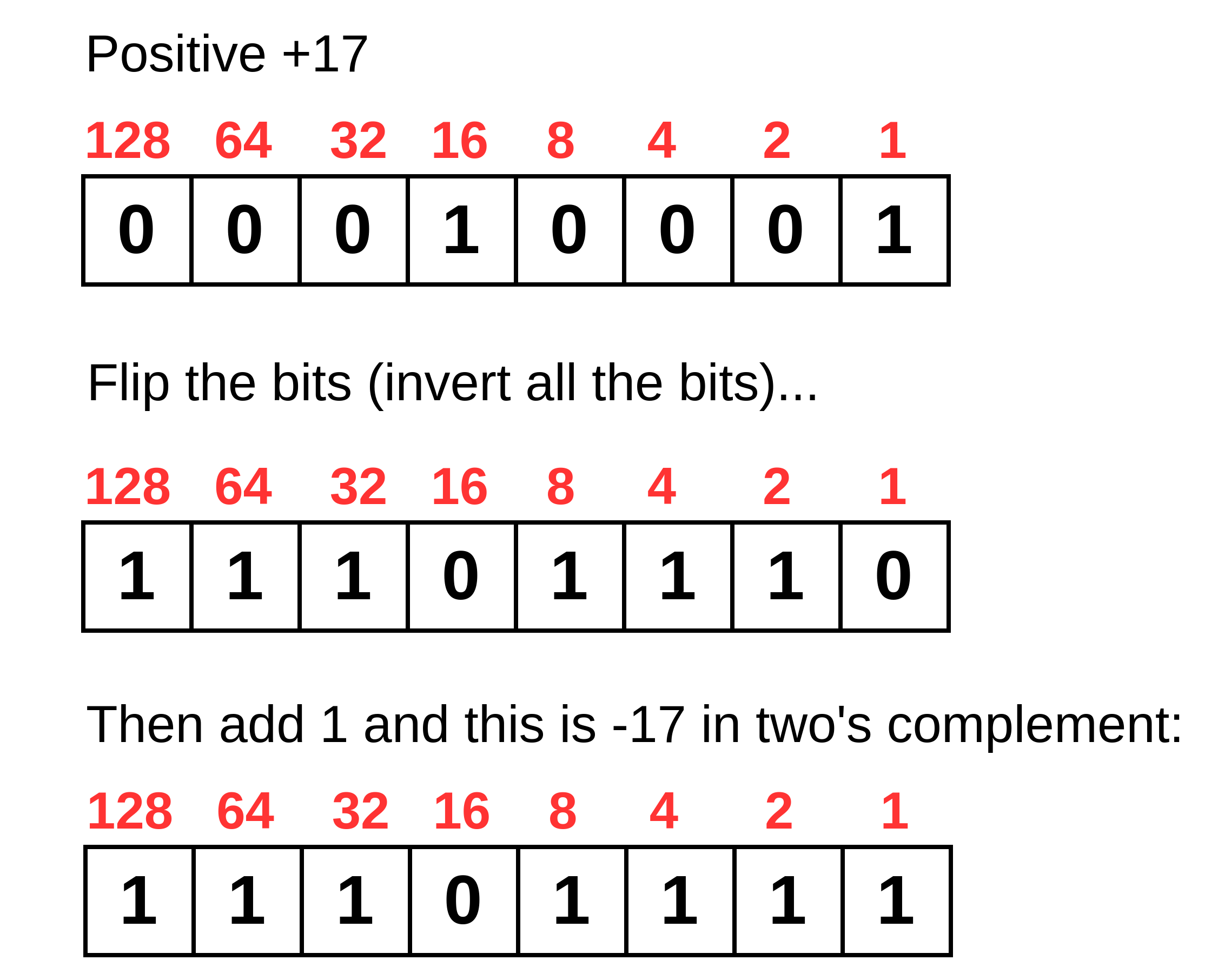
And to convert back again from -17 to +17, we just do the same procedure again:
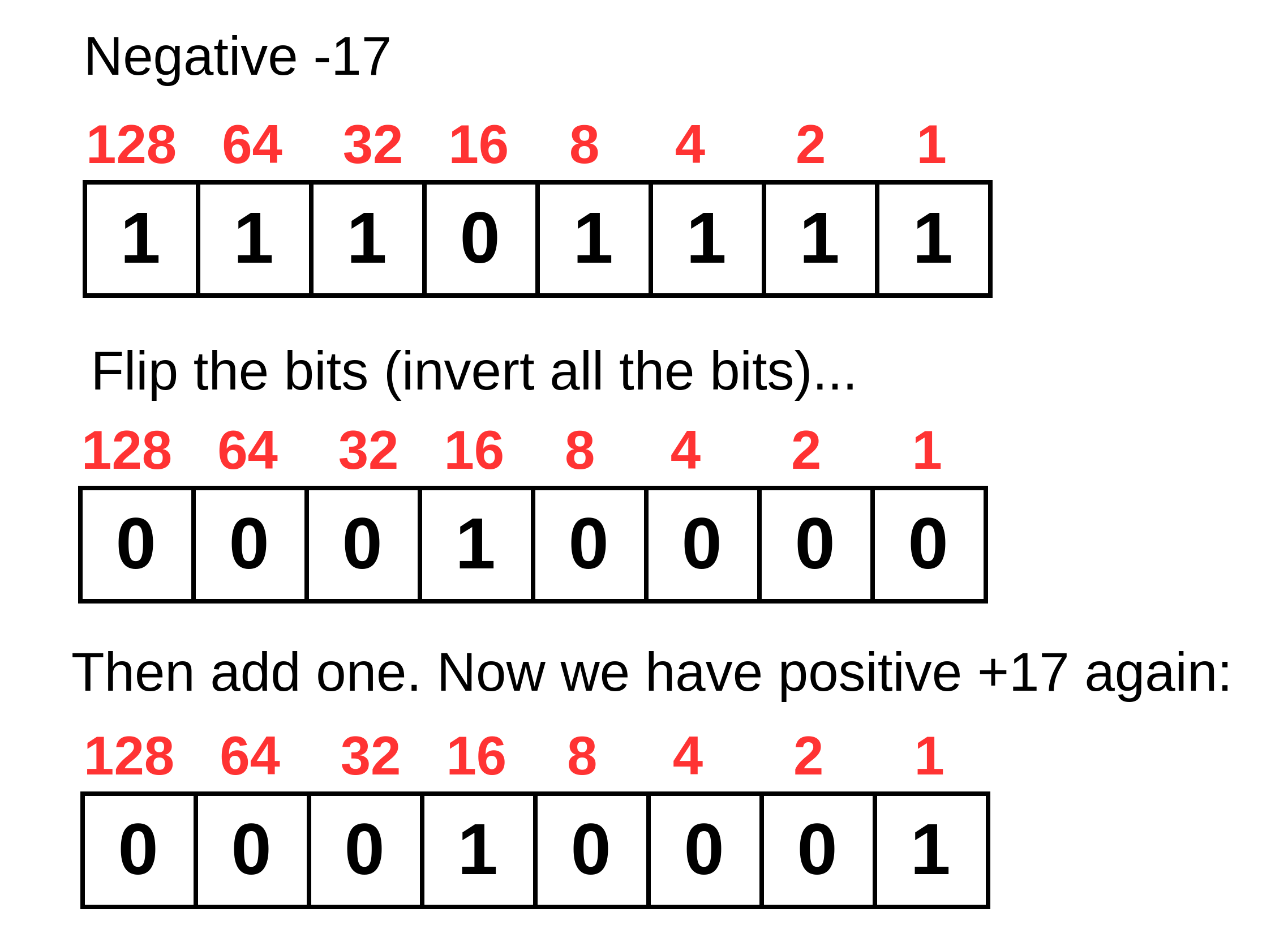
Simply flipping the bits would be a reasonable method of representing negatives on it’s own (this is called the one’s complement). So why do we subsequently need to add one? One of the key reasons we do this is to avoid the problem of ending up with two forms of zero: a positive zero and a negative zero. In maths we prefer to just have the one kind of zero to deal with!
Why is Two’s Complement Used?
The benefits of the two’s complement scheme are:
- Positive numbers are represented in the usual expected binary form and are easy to read
- It is simple to check if a number is negative. All we need to do is examine the left-most bit. If this is set to 1 then the number is negative.
- The electronic circuit necessary to perform negation is easy to build
More Examples of Two’s Complement
Number | 8-bit Binary |
---|---|
127 | 01111111 |
4 | 00000100 |
3 | 00000011 |
2 | 00000010 |
1 | 00000001 |
0 | 00000000 |
-1 | 11111111 |
-2 | 11111110 |
-3 | 11111101 |
-4 | 11111100 |
-128 | 10000000 |
Next: Fixed Point Numbers